
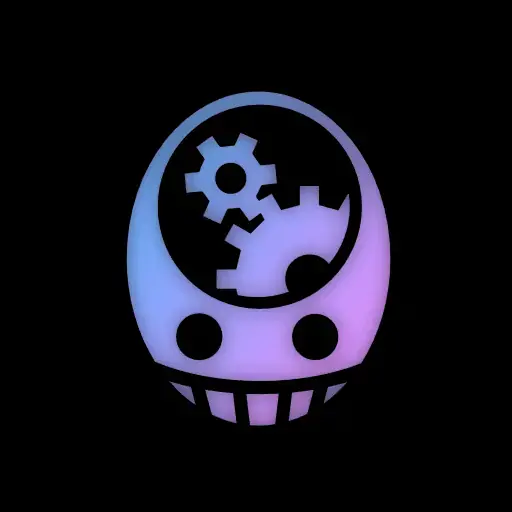
Using it in pipes looks cool. IMO the usage in writing git commit messages is actually not useful. Almost always you should be writing the why, not the what. Same thing for comments. Unless the code has a good reason to be written inscrutably e.g. for performance, write simple code and comment why you’re doing something as necessary. Which is not to say “the code comments itself”, but the “what” comments should be higher level at a function or file level
Russia can stop this at any time by just not invading them