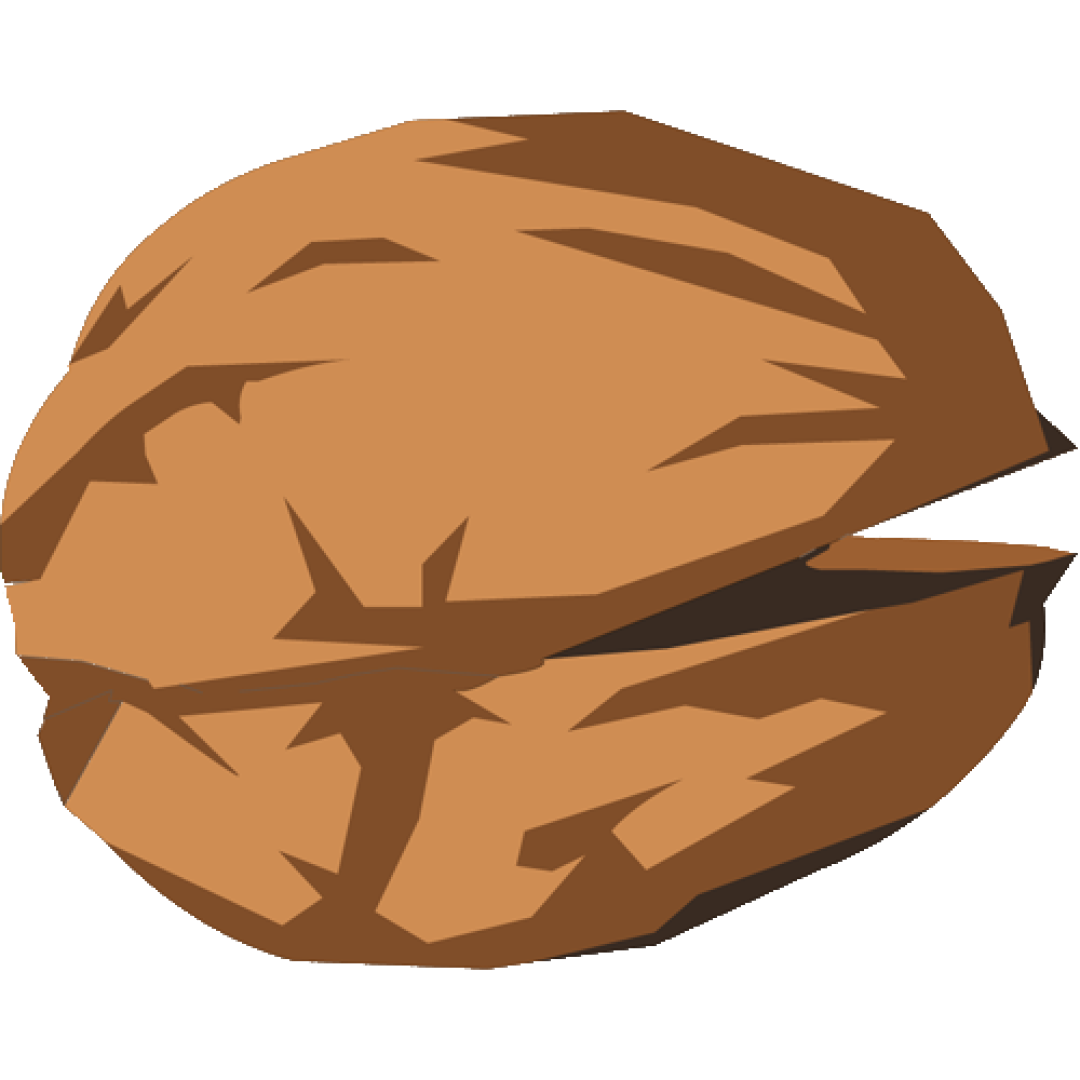
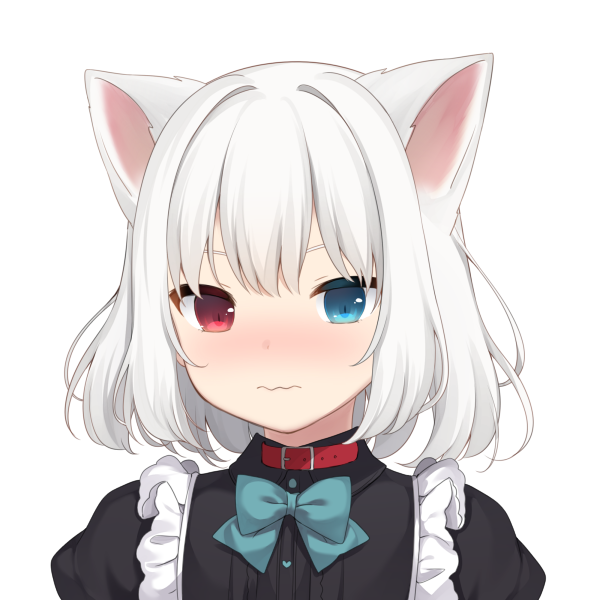
I mean yeah, but why? Like what did you like about it?
I mean yeah, but why? Like what did you like about it?
Claymore (the end was kinda mid)
Genuinely curious - why do you like it? I see this at the top anime of all time. I watched it a few years ago and i thought it was absolutely horrible. Like 2 or 3 out of 10.
I feel like the only reason i can see is “the main character is a bad guy” but that doesnt excuse trope-y terrible writing, flat characters, and mid-2000’s animation that aged horribly. Am i missing something?
Honestly, it’s because a bunch of programs i used disappointed me (performance, functionality, [being a web app at all], etc.) and i figured it couldnt be that hard to do it better. In some cases i was right, in most i was wrong. As it turns out though, I really like programming so i guess i’m stuck here
To be clear, fall through is implicit - when the case being fallen through is empty
That’s even worse… why isnt an empty case a syntax error?
I’m not sure I understand your point about fall through having to be explicit
As far as i understand it, every switch statement requires a break
otherwise it’s a compiler error - which makes sense from the “fallthrough is a footgun” C perspective. But fallthrough isnt the implicit behavior in C# like it is in C - the absence of a break
wouldnt fall through, even if it wasnt a compiler error. Fallthrough only happens when you explicitly use goto
.
But break
is what you want 99% of the time, and fallthrough is explicit. So why does break
also need to be explicit? Why isnt it just the default behavior when there’s nothing at the end of the case?
It’s like saying “my hammer that’s on fire isnt safe, so you’re required to wear oven mitts when hammering” instead of just… producing a hammer that’s not on fire.
From what i saw on the internet, the justification (from MS) was literally “c programmers will be confused if they dont have to put breaks at the end”.
the ergonomics expected of modern languages.
As someone learning c# right now, can we get some of those “modern ergonomics” for switch statements 💀
I cant believe it works the way it does. “Fallthrough logic is a dumb footgun, so those have to be explicit rather than the default. But C programmers might get confused somehow, so break has to be explicit too”
I miss fallthrough logic in languages that dont have it, and the “goto case” feature is really sick but like… Cmon, there’s clearly a correct way here and it isnt “there is no default behavior”
Saying “non negotiable” doesnt actually hold up in small claims, nor against basic resistance in most cases.
Look up your local laws, in some places carpets must be replaced at the expense of the landlord every X years, or if there is any kind of damage (caused by regular wear and tear) that could be a trip hazard. Pictures from move-in, carpets not being replaced when you moved in, etc. all help your case.
Last place i lived, I spent 30 minutes arguing on the phone with my previous landlord over flooring and got my 700 dollars back. Turns out most of the time they only vaguely know the laws they’re quoting, so if you come with confidence, prep, and a willingness to take it to small claims, they’ll fold to save themselves the effort.
That depends on your definition of correct lmao. Rust explicitly counts utf-8 scalar values, because that’s the length of the raw bytes contained in the string. There are many times where that value is more useful than the grapheme count.
I think it was this issue. Looks like maybe it got fixed some time this year? Iunno, i’ll look into it at some point
I did not dismiss it, I said measure the performance yourself.
Then why does anyone ask anything? Just figure it out yourself. Oh you read a book or went to college? Why? Should have just reinvented computers yourself man. Taking advantage of collective knowledge is for suckers /s
This means that the code performance is highly dependent on runtime conditions, and needs to be measured in the place where it’s used.
How is that helpful for OP? For example, if his question was about rust i’d say “options 1 or 2 should be identical for speed”, but if it’s python i’d say “match statements are just chained if/else chains, so a direct array index would be faster”. For another example, in python attribute access is a function call. x.y
in a loop is slower than assigning z = x.y
outside of the loop and calling z
in the loop.
You can absolutely generalize and have rules of thumb for performance.
If they already measured, then they would know which one is faster, because they measured it.
Measurements can have unintuitive results based on the dataset used (which, for benchmarks, usually end up being artificial datasets). OP’s measurements may not have been consistent with their working understanding, thus they ask outside sources to confirm the truth. Idk why you cant just give them the benefit of the doubt and like… answer the question they actually asked? The explanations for “why” that accompany that answer can also be incredibly helpful.
And all of these optimizations are just as effective after you measure them to see if they’re needed, and they’re no longer premature.
That implies that these optimizations are harder than doing it the “mundane” way. They’re not.
Here’s a fun micro optimization for compiled languages: on modern CPUs
x = x * (arr[0] * arr[1])
in a loop has better performance characteristics than
x = (x * arr[0]) * arr[1]
even though they do the same thing (in short, it’s because of the data dependencies for out-of-order execution - compilers wont make this optimization automatically for floats). How much harder is it to write the first one compared to the second one? How much harder to read is the first compared to the second?
So why would you not just make the first one your default? Now all your future uses of that pattern will perform better, for no extra effort except the amortized cognitive fee of changing your default option.
Look at OPs question. Could the answer fall under a rule of thumb that they can apply as their default option for a scenario? I’m pretty sure it can. So who cares about “premature” or not?
The particular question asked by the OP is very very unlikely to have any significant performance impact at all, unless it’s in an extremely hot loop running millions of times per frame
So instead of answering their question, you assume it isnt impacting performance and they’re just asking for no reason?
I literally had this exact question about python like 8 months ago. I had a file parser that needed to process different chunks based on a tag. Performance was critical, several thousand files, each ~3mb), the tag dispatch happened about 100,000-300,000 times per file. The original was implemented with if/else. I switched it to match because i thought it was faster, it wasnt. I looked at a lot of threads with answers like yours until stumbling upon dictionary dispatch (i.e. key = tag, value = first class function to call on the tag’s data) and array dispatch.
That change alone was a 15% performance improvement.
You have no idea how their program works, what their hot loop is, if they’re just asking out of curiosity, whatever. Just answer their fuckin question my dude. Platitudes are a waste of everyone’s time.
Rule number 2: stop dismissing performance questions just because of something some guy said decades ago. Performance matters, learning about performance matters, and answers like yours dont help anyone.
Did they ask if they should optimize, or did they ask which one generates more performant assembly? Which one of those questions did you answer?
Maybe they already measured and already knows this is a bottleneck. Maybe they are curious if match statements are a slow abstraction (e.g. in python, it’s essentially a chain of if/else. In rust it’s often compiled to an indexable table). Maybe the given example code is only partially representative of the actual code this is being applied to.
It’s so irritating to look up performance-related questions when this answer is at the top (and middle, and bottom) of every thread. I swear half the reason every piece of modern software runs like shit is because nobody bothered to learn how to optimize and now everyone just parrots that phrase instead of saying “i dont know”.
There’s tons of little “premature” optimizations that you can do that arent evil. Choosing the right data structure (how random is the access? Are you using keys? Does it need to be sorted?). Estimating time complexity and load size (e.g. “i’m parsing [11 million | 2] files, i should probably [keep time complexity in mind | ignore time complexity completely]”). Structuring loops in a way that’s easy for compilers to auto-vectorize - usually it’s not any harder to read what the loop is doing, so why not do it right away?
Yes i’m bitter =(
Is pycharm’s semantic highlighting still kinda ass? That’s the biggest thing that stopped me from using it over vsc. As of like may this year i remember there still being active issue tracking for it.
Counterpoint, i didnt like the rust book at all (as an inexperienced self taught ~6 months to a year into learning python at the time). Programming Rust and Rust In Action were far better.
Obligatory shoutout to Qownnotes for being excellent, fully open source, and with owncloud integration.
15532 on-type format (, by adding closing ) automatically
Thank fucking god lmao. The PR specifically mentions the Some(
case too, which is exactly where i encounter this the most.
Overall very nice changes
You could say that about anything. Of course you have to learn something the first time and it’s “unintuitive” then. Intuition is literally an expectation based on prior experience.
Intuitive patterns exist in programming languages. For example, most conditionals are denoted with “if”, “else”, and “while”. You would find it intuitive if a new programming language adhered to that. You’d find it unintuitive if the conditionals were denoted with “dnwwkcoeo”, “wowpekg cneo”, and “coebemal”.
“Unintuitive” often suggests that there’s something wrong with the language in a global sense
I mean only if you consider “Intuition” to be some monolithic, static thing that’s also identical for everyone. Everyone has their own intuition, and their intuition changes over time. Intuition is akin to an opinion - it’s built up based on your own past experiences.
just because it doesn’t look like the last one you used — as if the choice to use (or not use) curly braces is natural and anything else is willfully perverse on the part of the language designer.
I don’t think it’s that deep. All people mean when they say it is that “[thing] defied my expectation/prior experience”. It’s like saying “sea food tastes bad”. There’s an implicit “to me” at the end, it’s obvious i’m not saying “sea food factually tastes bad, and anyone who says they like it is wrong or lying”.
Here’s the script. It’s nothing fancy, and iirc it only works for top level functions/classes. That means you still have to take care of attributes and methods which is a little annoying, but for simple stuff it should save a bit of time.
For downsides, i’d like to add that the lack of function overloading and default parameters can be really obnoxious and lead to [stupid ugly garbage].
A funny one i found in the standard library is in time::Duration
. Duration::as_nanos()
returns a u128, Duration::from_nanos()
only accepts a u64. That means you need to explicitly downcast and possibly lose data to make a Duration after any transformations you did.
They cant change from_nanos()
to accept u128 instead because that’s breaking since type casting upwards has to be explicit too (for some reason). The only solution then is to make a from_nanos_u128()
which is both ugly, and leaves the 64 bit variant hanging there like a vestigial limb.
I’m no rust expert, but:
you can use
into_iter()
instead ofiter()
to get owned data (if you’re not going to use the original container again). Withinto_iter()
you dont have to deref the values every time which is nice.Also it’s small potatoes, but calling
input.lines().collect()
allocates a vector (that isnt ever used again) whenlines()
returns an iterator that you can use directly. You can instead passlines.next().unwrap()
into your functions directly.Strings have a method called
split_whitespace()
(also asplit_ascii_whitespace()
) that returns an iterator over tokens separated by any amount of whitespace. You can then call.collect()
with a String turbofish (i’d type it out but lemmy’s markdown is killing me) on that iterator. Iirc that ends up being faster because replacing characters with an empty character requires you to shift all the following characters backward each time.Overall really clean code though. One of my favorite parts of using rust (and pain points of going back to other languages) is the crazy amount of helper functions for common operations on basic types.
Edit: oh yeah, also strings have a
.parse()
method to converts it to a number e.g.data.parse()
where the parse takes a turbo fish of the numeric type. As always, turbofishes arent required if rust already knows the type of the variable it’s being assigned to.